Integrating Gravity Forms with SendSquared: A Technical Overview
The following code integrates with the gform_after_submission action in Gravity Forms, which activates once a form has been submitted. It verifies if the form ID matches the desired one to be sent to SendSquared, and if it does, the code gathers the form’s information, arranges the API details, and sends it to the SendSquared API through wp_remote_post. The code also examines the response code to confirm that the information was successfully sent to SendSquared. In case of any errors, the code logs them.
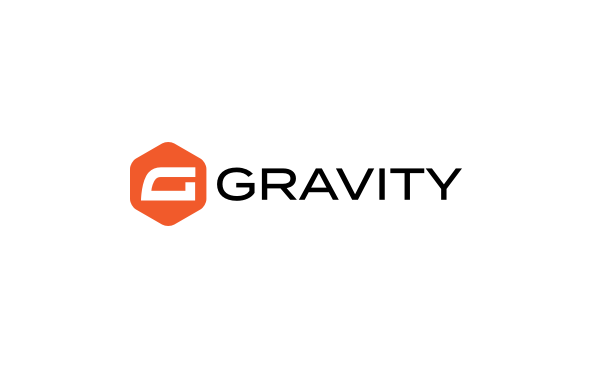
Note: You must substitute your-send-squared-token with the URL token of your intended SendSquared group to subscribe the user, and 123 with your form’s ID.
Example Code:
<?php
add_action( "gform_after_submission", "post_to_send_squared", 10, 2 );
function post_to_send_squared( $entry, $form ) {
// Only proceed if the form ID is 123
if ( $form['id'] != 123 ) {
return;
}
// API token
$token = "";
// Form data
$email = rgar( $entry, "your-email-from-a-form-here" );
$fname = rgar( $entry, "your-first-name-from-a-form-here" );
$lname = rgar( $entry, "your-last-name-from-a-form-here" );
$phone = rgar( $entry, "your-phone-from-a-form-here" );
// API URL
$api_url = "https://app-api.sendsquared.com/v1/pub/popup?token=" . $token;
// API data
$api_data = [
"email" => $email,
"phone" => $phone,
"first_name" => $fname,
"last_name" => $lname,
];
// Send the data to the API
$response = wp_remote_post( $api_url, [ "body" => $api_data ] );
// Check the response status
if ( is_wp_error( $response ) || wp_remote_retrieve_response_code( $response ) != 200 ) {
error_log( "Error submitting form to SendSquared: " . print_r( $response, true ) );
}
}
If you have any additional questions, feel free to reach out to SendSquared support.
Leave a Reply