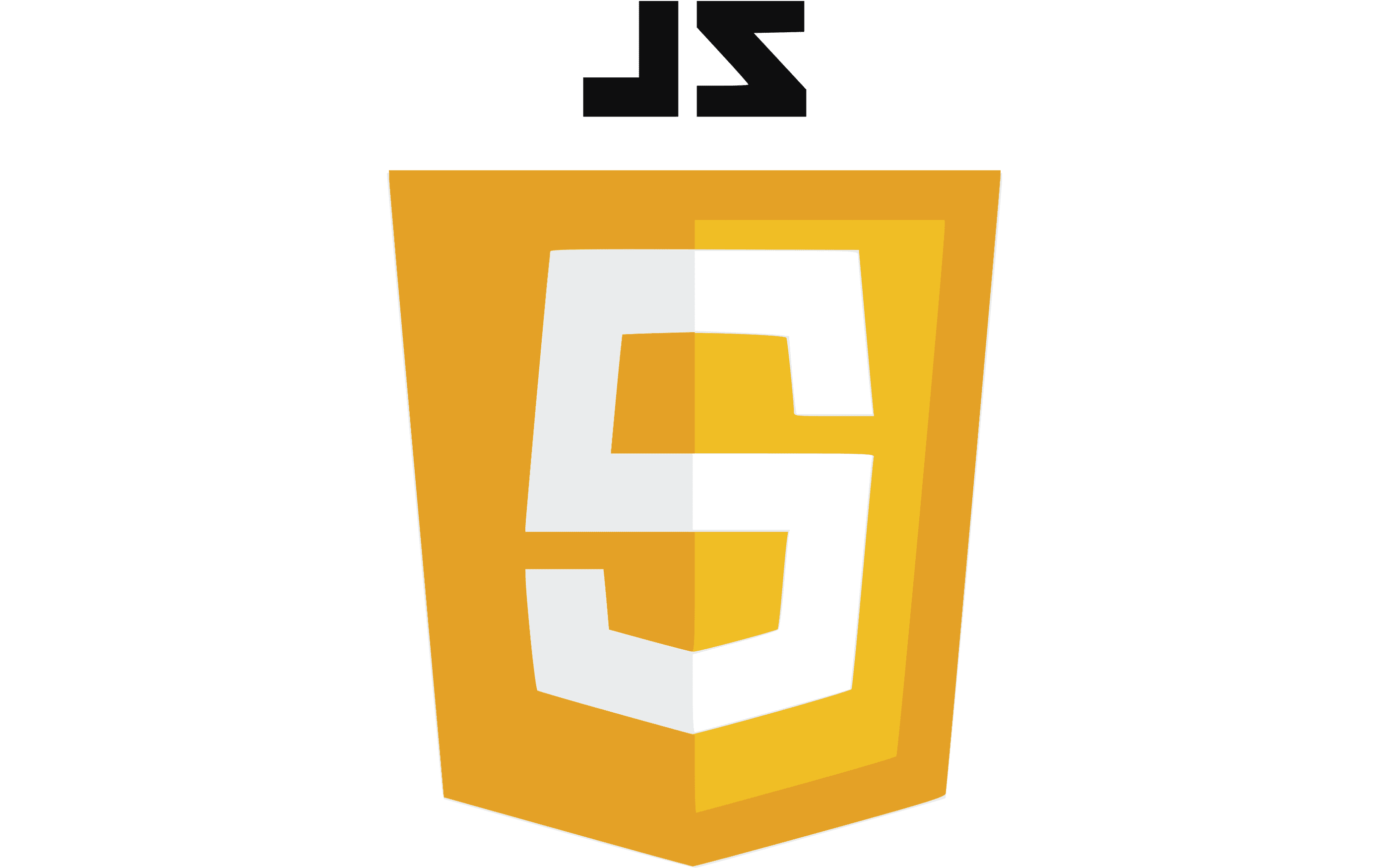
Add a Contact to SendSquared with Native JavaScript
Are you looking for a way to integrate your booking processes, or Booking Engine with SendSquared API to automate your lead generation process for Cart Amendment? If yes, you’re in the right place! In this tutorial, we’ll show you how to use native JavaScript to add a custom form submission function to your Booking page that sends data to SendSquared API using HTTP POST.
Here’s what you’ll need to get started:
- A SendSquared account with an active API key
- A Webflow account with a published website
- Basic knowledge of HTML and JavaScript
Ready to get started? Follow these steps:
- Add the Form Fields to Your Booking Page
Open your Booking Engine and add the form fields you want to collect data from. Make sure you give each field a unique name that matches the keys in your SendSquared API data object.
- Add JavaScript Function to Custom Footer section of your Booking Engine.
Go to your Booking Engine and add the new JavaScript function to the Custom Footer section. This is also commonly the place where you can add Google Analytics or similar tracking tools from Facebook/Meta and others. Once found, copy and paste the code below into the file:
function sendFormToSendsquared() {
// Get the form data
const formData = new FormData(document.querySelector('#your-form-id'));
const email = formData.get('your-email');
const phone = formData.get('your-phone');
const name = formData.get('your-name');
// Set the API URL with the token
const token = 'your-list-group-uuid';
const apiUrl = `https://app-api.sendsquared.com/v1/pub/popup?token=${token}`;
// Set up the API data
const apiData = { email, name, phone };
// Use fetch to send the data to the API
fetch(apiUrl, {
method: 'POST',
body: JSON.stringify(apiData),
headers: {
'Content-Type': 'application/json'
}
})
.then(response => {
if (!response.ok) {
throw new Error(`Network response was not ok (${response.status})`);
}
return response.json();
})
.then(data => {
console.log('Success:', data);
})
.catch(error => {
console.error('Error:', error);
});
}
document.querySelector('#your-form-id').addEventListener('submit', (event) => {
event.preventDefault();
sendFormToSendsquared();
});
Make sure to replace the “your-list-group-uuid” placeholder with your actual SendSquared API token. This code assumes that your form has two input fields with the name
attributes set to your-email
, your-phone
and your-name
.
- Add the Form ID to the JavaScript Code
Replace “your-form-id” with the ID of your form element in the document.querySelector()
method in the JavaScript code.
- Save and Publish Your Changes
Save the JavaScript file and publish your Booking Engine. Your lead form submission data should now be sent to SendSquared API when the form is submitted.
That’s it! You’ve successfully integrated your lead generation for Cart Abandonment submissions with SendSquared API using native JavaScript. If you have any questions or run into any issues, feel free to reach out to SendSquared support support@sendsquared.com for assistance.
Leave a Reply