March 3, 2023
Nicolas Wegener
Integrating Caldera Forms with SendSquared: A Technical Overview
This code hooks into the caldera_forms_submit_return filter in Caldera Forms, which is triggered after a form is submitted. It collects the form data, sets up the API data, and uses wp_remote_post to send the data to the API. The code also checks the response code to ensure that the data was successfully sent to the API. If there is an error, it logs the error.
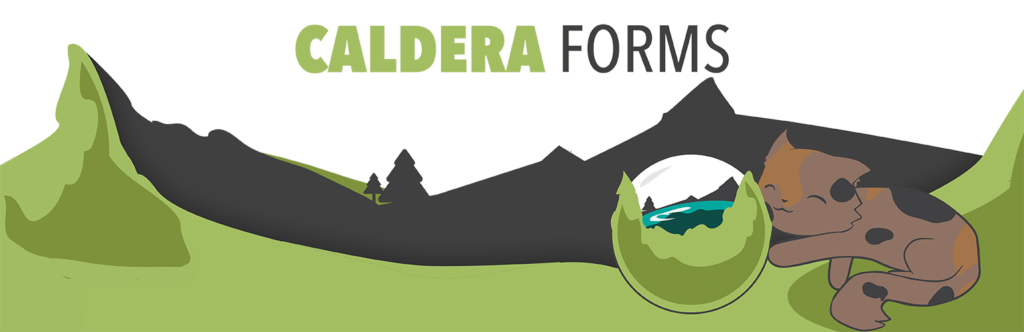
Note: You will need to replace your-email-field-id and your-name-field-id with the actual field IDs for the email and name fields, respectively, and your-list-group-uuid with the actual UUID of the list group the user will subscribe to.
add_filter( 'caldera_forms_submit_return', 'custom_form_submission', 10, 3 );
function custom_form_submission( $form, $referrer, $process_id ) {
// Get the form data
$email = $_POST['your-email-field-id'];
$name = $_POST['your-name-field-id'];
// Set the API URL with the token
$token = "your-list-group-uuid";
$api_url = "https://app-api.sendsquared.com/v1/pub/popup?token=$token";
// Set up the API data
$api_data = array(
"email" => $email,
"name" => $name,
);
// Use wp_remote_post to send the data to the API
$response = wp_remote_post( $api_url, array(
"body" => $api_data
) );
// Check the response code
if ( is_wp_error( $response ) || wp_remote_retrieve_response_code( $response ) != 200 ) {
// Log an error
error_log( "Error submitting form: " . print_r( $response, true ) );
}
// Return the form
return $form;
}
If you have any additional questions, feel free to reach out to SendSquared support.
Leave a Reply